The Game Board#
Layout#
The map is a 14x14 grid, including the outer walls. Both MOB-BOTs for either team will be on the same map. The only thing separating them is the walls of The Quarry. However, if the two MOB-BOTs meet, it could be an explosive battle!
NOTE: Two MOB-BOTs cannot stand on the same tile. However, MOB-BOT can stand on Traps, Dynamite, and Ores.
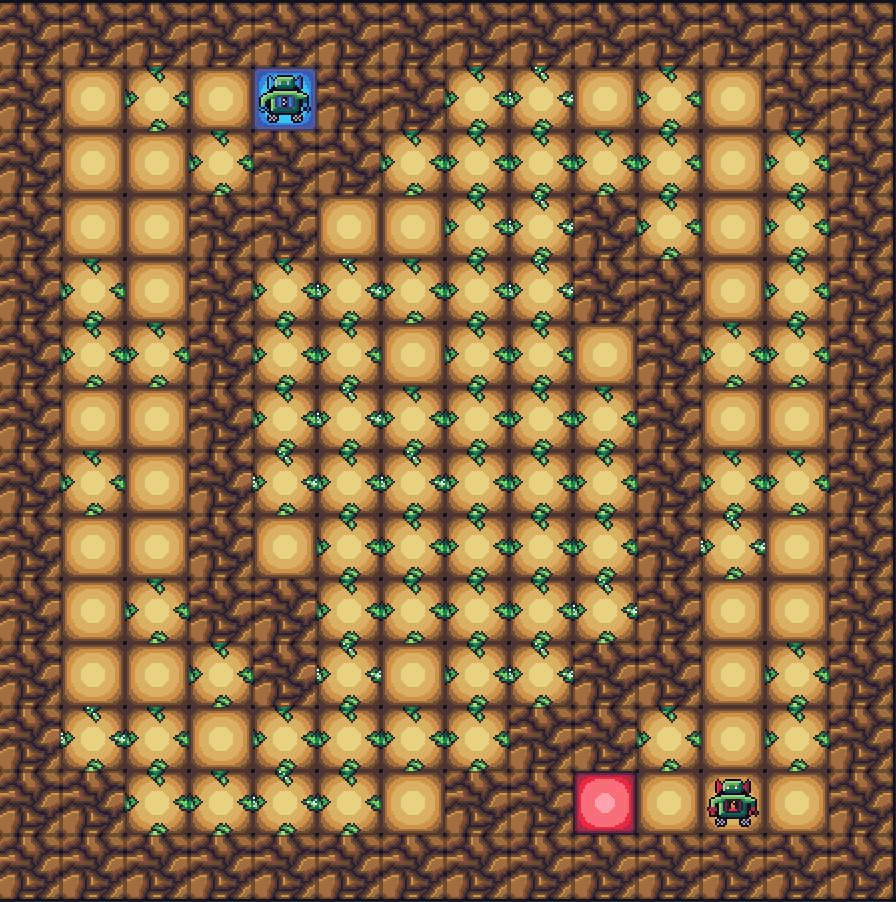
Tiles#
Coordinates#
The map is made of a 2D array of Tile objects. To access any tiles, use (y, x) coordinates like so:
world.game_map[y][x]
For example, looking at the game map, there is Copium ore close to the Church base at coordinate (2, 1) (remember that the top left is (0, 0)). To access that tile, type the following:
world.game_map[1][2]
Typing this will return the Tile object that has the ore at the specified coordinate.
Occupied_by#
Each Tile object – along with some others – has an attribute called occupied_by
. This attribute allows for each Tile
to act like a stack to see everything that is on top of it.
For example, assume a Tile object has an OreOccupiableStation (an object that gives you ores when you mine), Dynamite, and a MOB-BOT on it in that order. To see the first object the Tile is occupied by, type the following:
ore_occupiable_station = tile.occupied_by
Since the OreOccupiableStation is the first object in the stack, it will be the returned.
To check if the returned OreOccupiableStation is occupied by something, there are two ways to check this:
dynamite = ore_occupiable_station.occupied_by
dynamite = tile.occupied_by.occupied_by
Both of these would return a Dynamite object in this example. For every object that is on a Tile, you can either use
an object reference and call occupied_by
if it has it, or you can chain call occupied_by
on the initial Tile
object.
To access the MOB-BOT from the tile, you can do the following:
avatar = dynamite.occupied_by
dynamite = tile.occupied_by.occupied_by.occupied_by
Is_occupied_by and get_occupied_by#
If you simply want to check if a Tile is occupied by a certain object, you can type the following:
tile.is_occupied_by_object_type(ObjectType.EXAMPLE_OBJECT_TYPE)
The method will return a boolean representing if the Tile is occupied by the given enum. The method will only take an ObjectType enum.
Lastly, to receive an object that is on a Tile, type:
tile.get_occupied_by(ObjectType.EXAMPLE_OBJECT_TYPE)
This method will take an ObjectType enum and search for it. If found, it will return the object. Otherwise, the None
value will be returned.
The Placeables page will explain everything that can be placed on the map by MOB-BOT, and Enums will have the enums needed for every object that can be on the game map.
OreOccupiableStation#
OreOccupiableStation is a class that helps store the Ores and Ancient Tech. If you would like to check if a Tile has any Ore or Ancient Tech, type the following:
tile.is_occupied_by_object_type(ObjectType.ORE_OCCUPIABLE_STATION)
If this method returns True
, that means that either Copium, Lambdium, Turite, or Ancient Tech is on that Tile.
If there is an OreOccupiableStation object, you can do the following to check the type of the stored material:
ore = tile.get_occupied_by(ObjectType.ORE_OCCUPIABLE_STATION).held_item
ore.object_type == ObjectType.TURITE
NOTE: Calling get_occupied_by
may return a None
value, so you will need to incorporate null handling.
In this example, the code is checking if the ore is Turite. Comparing the ore’s ObjectType to the specified enum will return a boolean value indicating if it is the ore specified.
Below is all the ways you can check for all materials assuming
ore = tile.get_occupied_by(ObjectType.ORE_OCCUPIABLE_STATION).held_item
returned an object. Make sure to type these
verbatim.
ore.object_type == ObjectType.COPIUM
ore.object_type == ObjectType.TURITE
ore.object_type == ObjectType.LAMBDIUM
ore.object_type == ObjectType.ANCIENT_TECH
Bases#
Each team will have a base on the map. Their base will match their MOB-BOT and company color.
- Church Inc.
Church Inc. will have the blue base in the top left corner.
- Turing Co.
Turing Co. will have the red base in the bottom right corner.
When you want to cash in ores for points or upgrade your MOB-BOT, you can only do so at your base. Once MOB-BOT is standing on their base at the end of the turn, it will automatically deposit all the ores in its inventory.
More information on ores is found in Ores & Ancient Tech, and information on MOB-BOT is found in MOB-BOT.
Mining Interactions#
After mining ore from a Tile, there is a chance a new piece of ore is discovered underneath!
NOTE: As you move towards the center of the Quarry, the chance for Lambdium, Turite, and Ancient Tech to spawn significantly increases!
Here’s what can appear after mining specific ores:
Mined Ore |
Next Generated Ore |
---|---|
Copium |
Lambdium | Turite | Ancient Tech | Nothing |
Lambdium |
Ancient Tech | Nothing |
Turite |
Ancient Tech | Nothing |
Ancient Tech |
Nothing |
Visit Ores & Ancient Tech for more information on ore values.